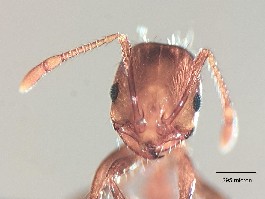
It provides a very simple API to commit and rollback transactions, and on top of that a UNIX-alike set of functions to perform most common operations (open(), read(), write(), etc.) in a non-intrusive threadsafe and atomic way, with safe and fast crash recovery.
The library guarantees file integrity even after unexpected crashes, never leaving your files in an inconsistent state.
On the disk, the file you work on is exactly like a regular one, but a special directory is created to store in-flight transactions.
It's written in C and has no dependencies on external libraries. It's based on the POSIX/Single UNIX Specification API, and it runs in all major UNIX variants. When available, Linux-specific system calls are used for improved performance.
It also comes with Python (both 2 and 3) bindings that provide a file-like object.
It's in the public domain, so you can link the library with anything you want.
Documentation
- For programmers using the library:
- Programmer's guide (start here)
- Public API reference
- Implementation documents:
The rest of the documentation, including manpages, can be found in the source.
Code samples
Using the UNIX-alike API (simplified, without error checking):char buf[] = "Hello world!"; jfs_t *file; jtrans_t *trans; file = jopen("filename", O_RDWR | O_CREAT, 0600, 0); jwrite(file, buf, strlen(buf)); jclose(file);
Using the basic, lower-level API (simplified, without error
checking):
char buf1[] = "Hello "; char buf2[] = "world!"; jfs_t *file; jtrans_t *trans; file = jopen("filename", O_RDWR | O_CREAT, 0600, 0); trans = jtrans_new(file, 0); jtrans_add_w(trans, buf1, strlen(buf1), 0); jtrans_add_w(trans, buf2, strlen(buf2), strlen(buf1)); jtrans_commit(trans); jtrans_free(trans); jclose(file);
Using the Python bindings:
import libjio f = libjio.open("filename", libjio.O_RDWR | libjio.O_CREAT) t = f.new_trans() t.add_w("Hello world!", 0) t.commit()
More complete code samples are included with the source.
Download
The current version is 1.02 (released on 2011-02-27): You can also browse all released files.Patches are welcome. The source code is managed using git. You can browse the repository, or clone it by running:
git clone https://blitiri.com.ar/repos/libjio